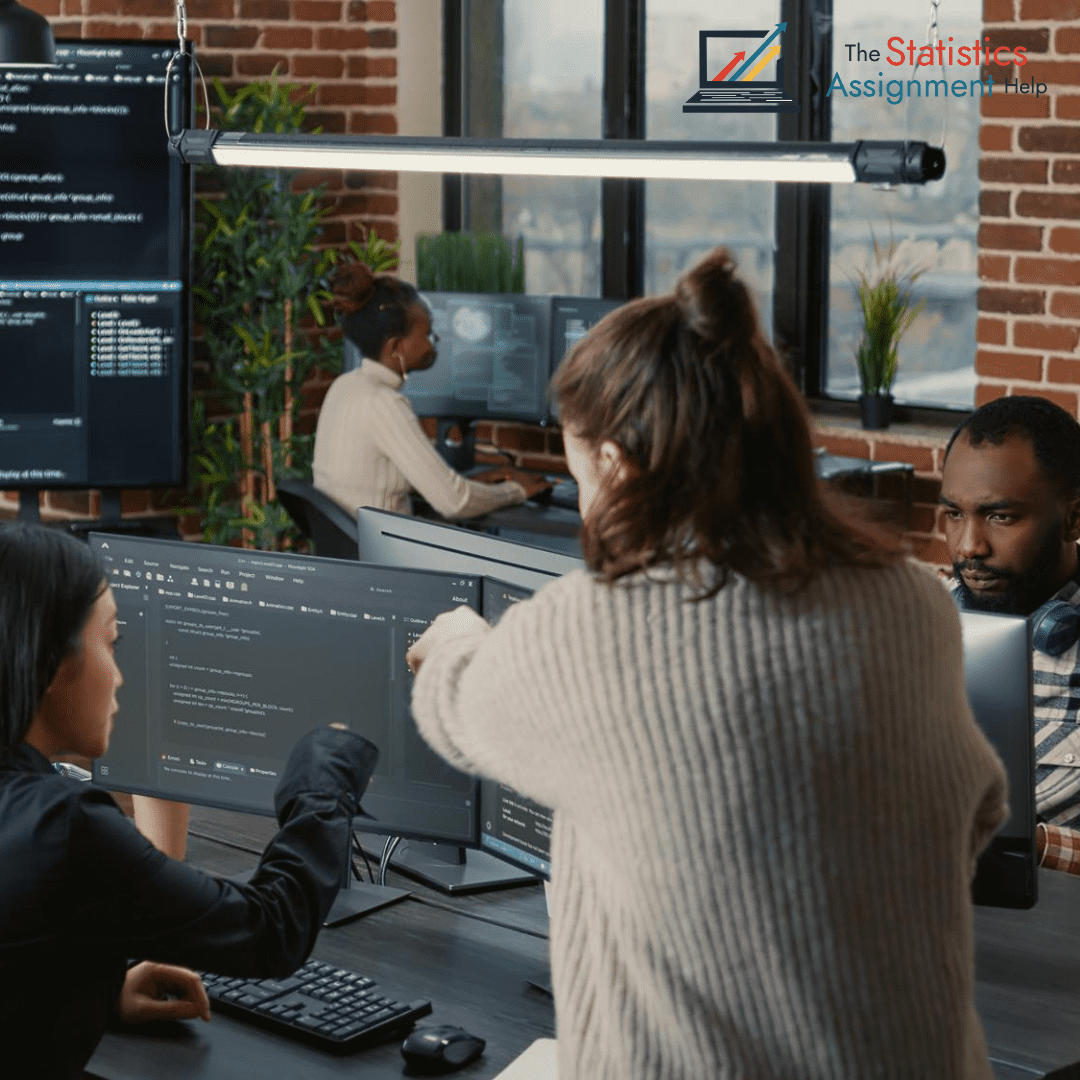
- 11th Nov 2022
- 06:03 am
1. Recursion Task
def sumofx(x): # defining the function name for recursion with a number as a parameter
if x == 1: # if the number is 1 return the number
return x;
else:
return x + sumofx(x - 1) # here recursion is used as the function is calling itself again and again until the number is reduce to 1
print("sumofx(15)")
print(sumofx(15)) # calling the function sumofx with 15 passed as parameter
print("sumofx(2)")
print(sumofx(2)) # calling the function sumofx with 2 passed as parameter
print("sumofx(5)")
print(sumofx(5)) # calling the function sumofx with 5 passed as parameter
print("sumofx(17)")
print(sumofx(17)) # calling the function sumofx with 17 passed as parameter
2. Guest Objects Task
class Guest: # class name
def __init__(self, first_name, last_name, phone, room): # constructor with first name,last name, phone and room no passed as argument
self.first_name = first_name # assinging value to first name
self.last_name = last_name # assinging value to last name
self.phone = phone # assinging value to phone
self.room = room # assinging value to room
self.num_of_rooms = 20 # initalising value 10 to num of rooms
def get_room(self): # this method return the number of rooms
return self.room
def set_room(self, value): # this method is used to set the value of number of rooms
if self.num_of_rooms < value>
print("Rooms greater than 20")
self.room = 0
else:
self.room = value
def get_guest_details(self): # this method return the details of the guest in a string manner
return self.first_name + " " + self.last_name + ", " + str(self.phone) + ", " + str(self.room)
guestObj = Guest("ABC", "XYZ", 8856254125, 15) # creating a object of class guest using the constructor
print(guestObj.get_guest_details()) # printing the guest details using the get_guest_details() method
guestObj.set_room(25) # setting the number of rooms
guestObj.set_room(2) # setting the number of rooms
print(guestObj.get_guest_details()) # printing the guest details using the get_guest_details() method